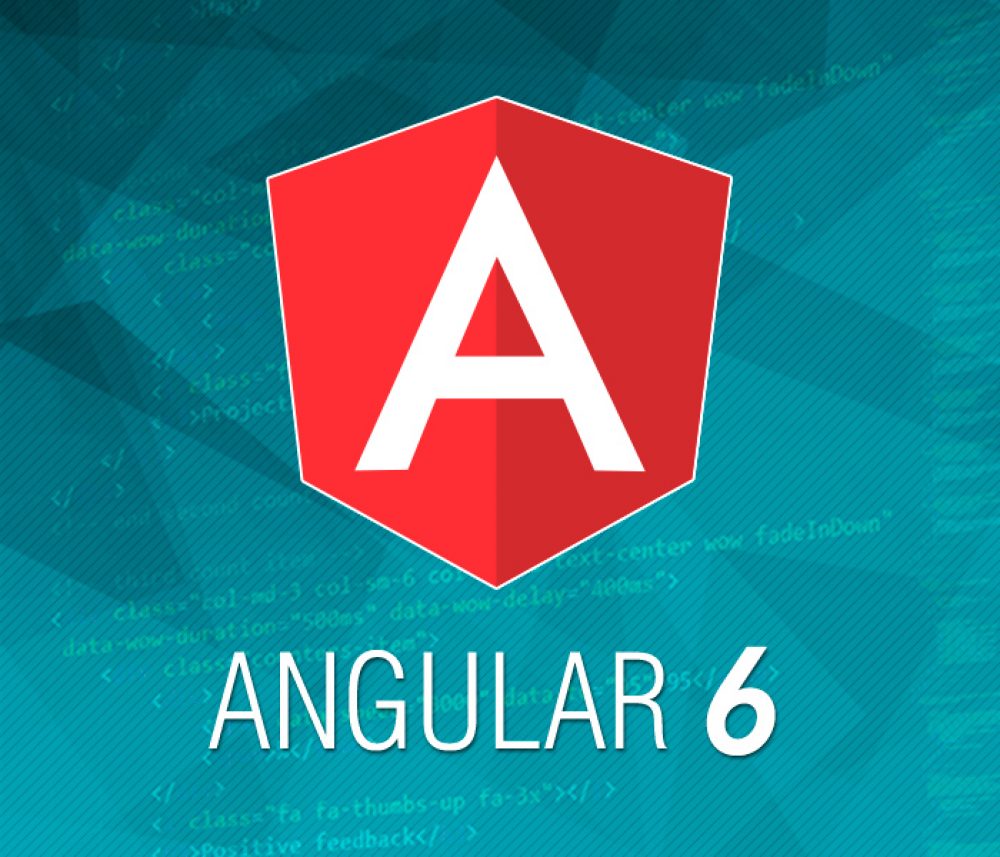
【NGX-Charts】超絶素晴らしいAngular6対応のグラフライブラリがあるからみんなに知ってほしい
はじめに
どうも、福岡のmeです。
最近はAngular6を使ったSPA開発をメインにやっているのですが、
ダッシュボードにグラフを出したいという要件があったので、何か簡単の実装できるツールはないものかと探していた時に
”これは・・!”というものを見つけたのでご紹介したいと思います。
自分が探していたものは以下の条件に合うものでした。
- Angular6 に対応していること
- 棒グラフ、パイ、線グラフなど、種類がいくつかあるもの
- 使い方がシンプルなもの
今回使用するライブラリ
まず目を引いたのはおしゃれなデザインのグラフたちです。
こんなグラフがダッシュボードに置いてあったらテンションが上がって、仕事により一層身が入りそうです。
ドキュメントも読みやすく、それぞれにサンプルコードがついておりさくさく試すことができました。
グラフの種類も豊富で、様々な種類があるようです。
今回はこの中からオーソドックスな棒グラフとパイを試して見ました。
環境
私のAngular環境は以下の通りです。
Angular CLI: 6.0.8 Node: 8.11.2 OS: darwin x64 Angular: 6.0.7 ... animations, common, compiler, compiler-cli, core, forms ... http, language-service, platform-browser ... platform-browser-dynamic, router Package Version ----------------------------------------------------------- @angular-devkit/architect 0.6.8 @angular-devkit/build-angular 0.6.8 @angular-devkit/build-optimizer 0.6.8 @angular-devkit/core 0.6.8 @angular-devkit/schematics 0.6.8 @angular/cli 6.0.8 @ngtools/webpack 6.0.8 @schematics/angular 0.6.8 @schematics/update 0.6.8 rxjs 6.2.1 typescript 2.7.2 webpack 4.8.3
インストール
npmを使います。
npm install @swimlane/ngx-charts --save
app.module.tsに追加
import { BrowserModule } from '@angular/platform-browser'; import { NgModule } from '@angular/core'; import { AppComponent } from './app.component'; import { NgxChartsModule } from '@swimlane/ngx-charts'; import { BrowserAnimationsModule } from '@angular/platform-browser/animations'; @NgModule({ declarations: [ AppComponent ], imports: [ BrowserModule, NgxChartsModule ], providers: [], bootstrap: [AppComponent] }) export class AppModule { }
アニメーションサポートが必要なので、BrowserAnimationsModuleも加えておきましょう。
インストール作業は以上です。
棒グラフを作成してみた
まずはグラフの設定と渡すデータを用意します。
import { Component } from '@angular/core'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { // グラフの表示サイズ view: any[] = [700, 400]; // 設定 showXAxis = true; showYAxis = true; gradient = false; showLegend = true; showXAxisLabel = true; xAxisLabel = '国'; showYAxisLabel = true; yAxisLabel = '人口'; // カラーテーマ colorScheme = { domain: ['#5AA454', '#A10A28', '#C7B42C', '#AAAAAA'] }; // サンプルデータ sampleData = [ {name: 'Germany', value: '100'}, {name: 'France', value: '200'}, {name: 'Japan', value: '300'} ]; constructor() { } onSelect(event) { console.log(event); } }
グラフに渡すデータは’sampleData’です。
app.component.tsで定義した設定をグラフに当てます。
<ngx-charts-bar-vertical [view]="view" [scheme]="colorScheme" [results]="sampleData" [gradient]="gradient" [xAxis]="showXAxis" [yAxis]="showYAxis" [legend]="showLegend" [showXAxisLabel]="showXAxisLabel" [showYAxisLabel]="showYAxisLabel" [xAxisLabel]="xAxisLabel" [yAxisLabel]="yAxisLabel""> </ngx-charts-bar-vertical>
アウトプットは以下の通りです。
棒グラフに渡すサンプルデータの量をちょっと増やして見ました。
このように指定したサイズ内で自動的に伸縮してくれます。
パイ
オーソドックスなパイチャートも作成してみました
<ngx-charts-pie-chart [view]="view" [scheme]="colorScheme" [results]="sampleData" [legend]="showLegend" [explodeSlices]="explodeSlices" [labels]="showLabels" [doughnut]="doughnut" [gradient]="gradient"> </ngx-charts-pie-chart>
import { Component } from '@angular/core'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { // グラフの表示サイズ view: any[] = [700, 400]; // 設定 showLegend = true; showLabels = true; explodeSlices = false; doughnut = false; // カラーテーマ colorScheme = { domain: ['#5AA454', '#A10A28', '#C7B42C', '#AAAAAA'] }; // サンプルデータ sampleData = [ {name: 'Germany', value: '100'}, {name: 'France', value: '200'}, {name: 'Japan', value: '300'} ]; constructor() { } onSelect(event) { console.log(event); }
アウトプット
Advanced Pie Chart
こちらは別デザインのパイチャートです。。
<ngx-charts-advanced-pie-chart [view]="view" [scheme]="colorScheme" [results]="sampleData" [gradient]="gradient"> </ngx-charts-advanced-pie-chart>
import { Component } from '@angular/core'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { // グラフの表示サイズ view: any[] = [700, 400]; // 設定 showLabels = true; explodeSlices = false; doughnut = false; showLegend = true; gradient = false; // カラーテーマ colorScheme = { domain: ['#5AA454', '#A10A28', '#C7B42C', '#AAAAAA'] }; // サンプルデータ sampleData = [ {name: 'Germany', value: '100'}, {name: 'France', value: '200'}, {name: 'Japan', value: '300'} ]; constructor() { } onSelect(event) { } }
アウトプットはこんな感じになりました。
まとめ
いかがだったでしょうか。設定部分のタグをいじるだけでいろんなグラフが簡単に作成できるライブラリのご紹介でした。
Angular用のグラフライブラリは結構出ているのですが、数年の間に何度もバージョンを重ねている
Angularに最新の状態で対応しているものが少なく、安定しているものを見つけるのは結構大変ですよね。。
Angularを使っている方はぜひ一度試して見られてはいかがでしょうか。
公式ドキュメントはこちらから。